As I mentioned in one of the original instructions for my first walkthrough on NATAS, I wanted to go at it with Python. My motivation for this was during my initial search on how to complete the objectives using common tools in Kali. While doing so I found a lot of walkthroughs using python. I also recently discovered the man John Hammond having an entire series on NATAS with Python. I recently started to devote some time to learning python so I can continue growing on this skill overtime as well.
Purpose:
To strengthen my skills using Python. I have recently finished a book titled “Python Crash Course, 2nd Edition“. This book has a three big projects to finish it off but instead I wanted to put the time towards NATAS. In the future I may go back and finish the projects listed in the book and maybe do a write up about it as well.
I am unsure yet if I will complete the entire NATAS wargame using python. So far I have only completed up to level 10. Today I am only writing about levels 1 through 5. While doing this project I do not plan on looking at any write ups or videos specific to NATAS. Instead, I would like to spend my time in documentation and other sources online forcing me to read and understand what it is I am doing. Rather then copying, pasting and moving on.
Level 0
Similar to the original walk-though there is not much to do on level 0. This is a good time to introduce the library and concepts we will use.

If we would like to execute each of the python files without specifying the python interpreter in bash we can declare it using the #!/bin/env python3. The env python3 may change on your system but is determined by the address as to where it is located on your local machine.
The next line we will import the requests library (see references).
To make it easier to read we will also define three string variables, url, user, upass. All of which are going to be used as parameters in the get function.
Next we will define r as the variable that stores get request.
r = requests.get(url, auth=(usr, upass))
Now understanding how parameters are passed to a function using arguments, I wanted to see the actual library defining requests.get. In order to find where the packages are stored you can run python3 in a terminal session. Then you can type help(‘modules’). This will give you an idea of where to start looking.
As you can see we are throwing the url and user/upass as paramters.
That is because the request function as seen below is looking for several arguments. (from what I understand the first argument “method” is defined with .get or .post etc..) The first argument the request function is looking for is the url. because we are passing it in order (inside our r variable) we do not need to define what we are passing. In other words if we were to move the url parameter to the right of auth we would have to define url=url. Similar to how anything after url will need its parameters defined with =. This will make more sense as I continue onward.
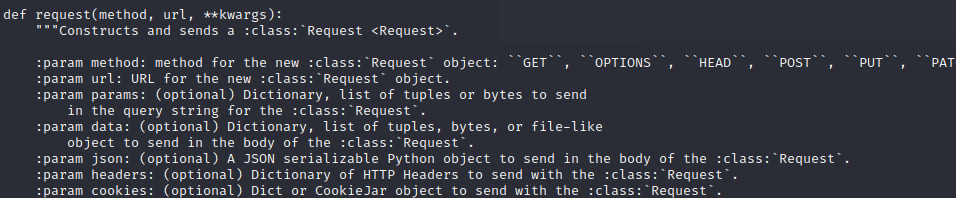
The last line is print (r.text). This print function is just displaying the text value of r (UTF-8). Essentially this is doing the same thing as if we were to view source code on Google Chrome or Firefox. We now have the password for Natas1.

Level 1
Now that we have the password for the next level, we will go ahead and copy this file and rename it to natas2.py. In natas2 we will modify the three variables, allowing access to natas1.

Run that and we will see the following.

If we were running this on the browser we would not be able to right click and view source. Instead we would of had to use the hot-key to gain access. Since we are using python we do not need much and already have the password for the next level.
Level 2
Next we will do the same thing. Copy, adjust variables and execute to view source.
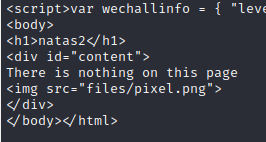
Fairly straight forward. With this source code we are able to see a directory called “files”. Lets go ahead and modify the .py file and visit this new directory. There you will find even more source code. Some of which is congested with html characters. I cleared this up a little by using html.unescape().

When visiting files directory you will see a reference to a users.txt. Lets go ahead and view that file by adjusting our url variable again. Also look below at how I defined the html.unescape(). This is imported with import html.
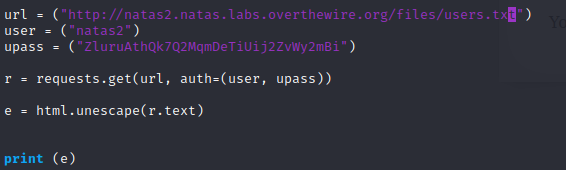
We will then see a password for natas3.

Level 3
Again, we will adjust variables to visit the natas3 page.

If you remember from my previous post this is referencing a robots.txt file. This file is used by search engines, giving access to certain directory’s and preventing others. I explained it better in my other walkthroughs a few months back if you would like to take another look. So if we were to visit /robots.txt, we would see the following.
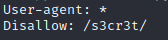
Lets take a look at this “/s3cr3t/” directory and see what we find in there.

We notice again a reference to a users.txt file. In there we will find the password for level 4!

Level 4
After adjusting the variables to access natas4, we will see the following.

As discovered last time, this particular line is referencing a particular header “Referer”. Without modifying this it is saying we are coming from Natas4. Instead we want it to say we are coming from Natas5.

Instead of using burp this time, we will just adjust our requests.get parameters. Alongside auth we will add headers. We will then define our custom headers. If not familiar with Python, we are essentially defining a dictionary inside of python. The referer being the key and the url being the value. All entered inside {}.


Level 5
Next we will get to play around with the cookies. Once logged into Natas5 we notice it say that we are not logged in.

Similar to the custom headers in Level 4 we will enter custom cookies. First we can print the cookies in python to see what is being requested.
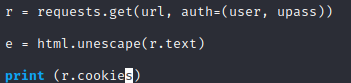

After executing the python file we found a cookie referencing loggedin=0. In other words it is saying false, the user is not logged in. Lets modify that to say 1.


Conclusion
I am really enjoying this project. I have messed around with python for some time now. I just recently had to brush up on somethings and wanted to go a bit further. Much of my experience was just simple functions to use at home. One also I planned to introduce in my work place but ended up passing on the opportunity for something else.
Tomorrow I will most likely finish out the writeup for the next 5 levels. From there I am not sure if I will continue through level 20 or if I will dedicate some time elsewhere. (I have some other good ideas with python to add to homelab) Either way I am having fun with it and I hope someone somewhere can take something away as well!