Purpose:
This lab will help strengthen the understanding of SQL injection. In this lab we will create a MySQL database, setup a simple Apache web server and then attack! This lab will continue over several write-ups and Youtube videos. Overtime we will use more advanced techniques to not only attack the web application but to harden it. This first series will be focused strictly on SQL injection. In the future I plan to dive further into web exploitation and have labs for each of them.
Prerequisites
Before starting this lab we will need to ensure the following packages are installed
1. apache2
2. php
3. libapache2-mod-php
4. mysql-server
note: Depending on Linux distribution it may be installed in a number of ways. For example with Ubuntu we will use the Advanced Packaging Tool (apt) to install our packages.
example: sudo apt-get install apache2
We also want to ensure that once apache2 is installed that it is active
sudo systemctl status apache2
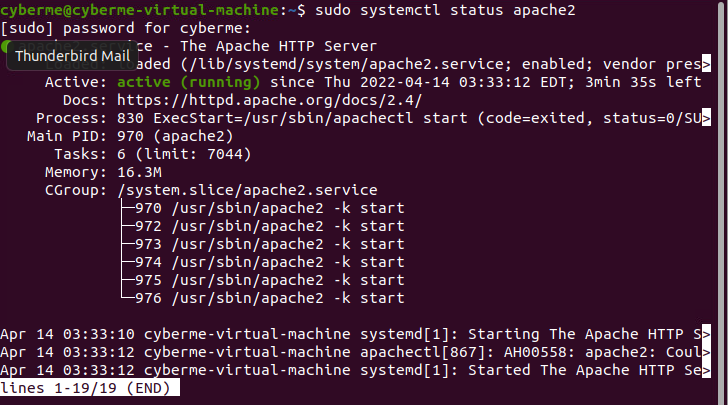
Another thing we can verify is if PHP is installed and that we have the correct version number. To do this lets head over to /var/www/html (explained in next block) and create an info.php file. Inside of the info.php file we will want the following:
<?php
phpinfo();
?>
Now we can view this page in a web browser by inputting the machine ip address that is hosting this file followed by the file name
example:
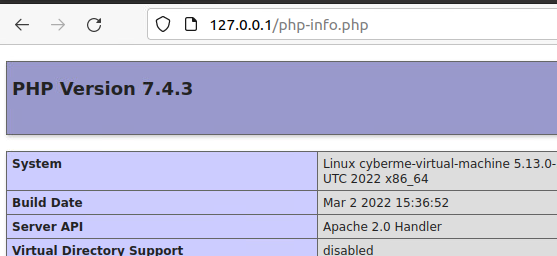
File Structure
As I mentioned above when looking into the info.php file, for this lab we will mostly be inside of /var/www/html. All of our .php and .html files will be located here. This is what apache2 web server uses in order to host its pages.
Creating a simple login form
Before diving into MySQL and the various PHP files we require, lets create a simple html page which will contain our login form.
- Navigate to /var/www/html and create login.html
touch login.html
- Inside of login.html we will want the following code
vim login.html
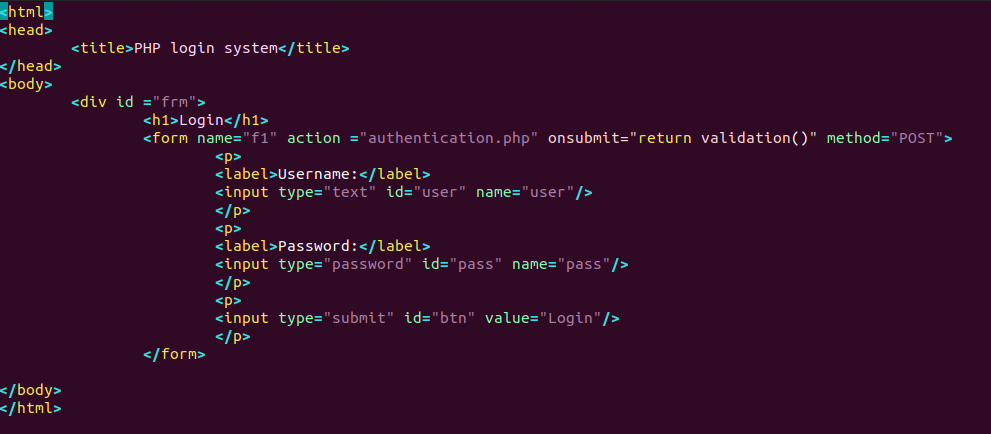
Most of this is self explanitory even for those that have not touched HTML. Biggest takeaway here would be the form’s action and method. From the method ‘post’, we can infer that we are sending data (login/password). From the action ‘authentication.php’, we now know where this information is going. Also the various id’s for the inputs are important to understand.
Configure MySQL
Next we will want to setup our MySQL database. First things first is using a simple built-in tool to setup MySQL. This will allow us to setup a root password along with disable/enable some other features.
sudo mysql_secure_installation
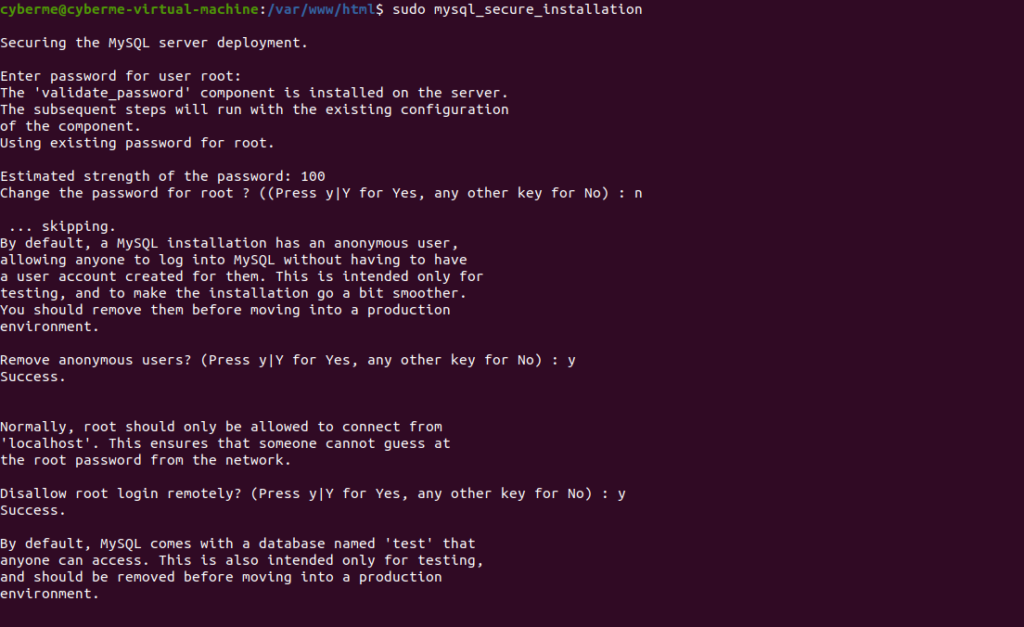
Next we will want to create a database to store our tables and data inside of. Before doing so we will want to connect to our MySQL server using our root login.
mysql -u root -p
In order for us to create the database we will use
CREATE DATABASE hackme;
We can then verify the database has been created by using SHOW
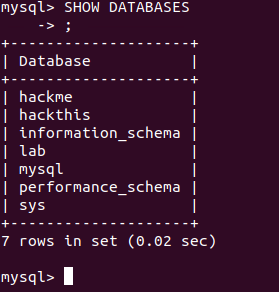
In order to store data into our database we need a table. First lets connect to our database
USE hackme;
The next command will be used to create/define our users table inside of the database we just connected to.
CREATE TABLE users (username VARCHAR(20), password VARCHAR(20));
The label username will be the first column restricted to 20 characters (string value) and same with password.
This can be verified by using DESCRIBE
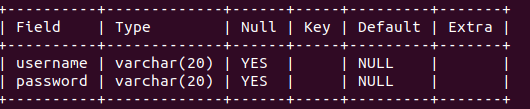
Now we will want to create some users and store them into our table.
INSERT INTO users (username,password)
VALUES("jack","1234");
We can then create a simple query to select all from users
SELECT * FROM users
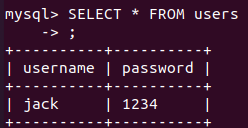
Connection.php
Next we will work on the connection.php file. This file will store the credentials to connect to our MySQL server. It will also be used for troubleshooting, giving us an error if we are unable to authenticate.
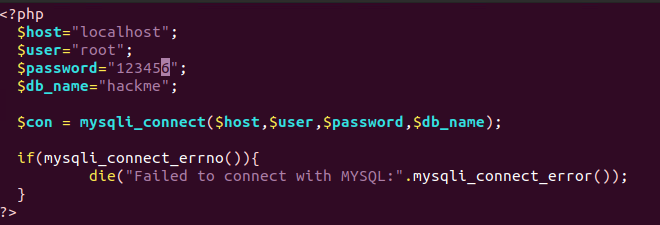
As you can see we have the variables defined at the top storing not only the credentials but the host and database name. The $con variable is using the mysqli_connect function to pass in those parameters which will be passed to the next file we create. Finally is the built-in error-handling function at the bottom, giving us an error if we are unable to connect. So if we were to navigate to this page on our machine and we were unable to authenticate for whatever reason you would see the following:

If we were able to authenticate we would have a blank screen.
Authentication.php
Finally we will create the authentication.php file. This is where the magic happens.
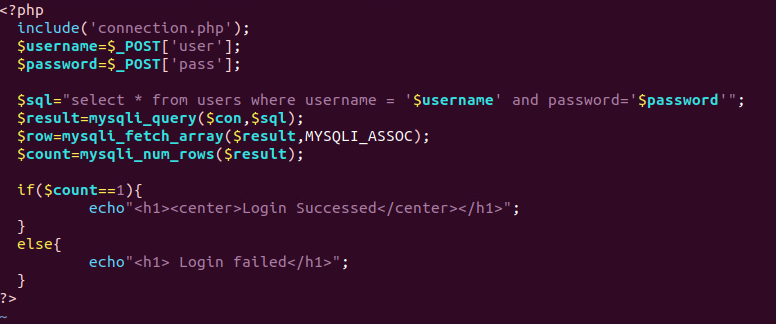
At the very top you will see the connection.php included. Just below that you will see two new variables being defined. $username and $password which are being assigned to the values from post method on login.html. $sql is the SQL query that will be used to search our table. This will be better explained in the next write up.
$result is using the mysqli_query function to pass the $con variable from connection.php (host/credentials/database) for the first argument, followed by the SQL query for the next.
$row is using the mysqli_fetch_array function which essentially would fetch one row of data from result set and return it as an array. Not necessary in this lab but may be used later on.
$count is using the mysqli_num_rows function which will return the number of rows in result set. Essentially, if parameters from SQL query match result set, count will be assigned a 1. If no matches are found then it will be null.
Lastly is the if statement at the bottom. This statement only uses the count variable we just discussed to determine if the credentials passed on the login form match any credentials in the database. If so then it will echo Login Success. Do not ask me why I have Successed in the picture, I am not going back and fixing it either. If null, the else statement will be used to print Login failed.
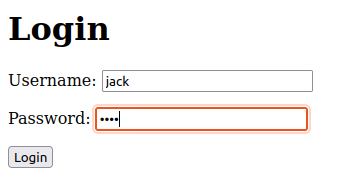

Conclusion
We now have a simulated website that we can exploit. This will allow us to not only learn more about SQL injection but to put it in practice. The hacking portion of this lab will be covered in detail in the next discussion later this week. I hope enough detail is here in this instruction to get you setup so you can follow along. Also in two weeks I plan to start recording some videos to cover this as well. Stay tuned.
I would also like to mention their are plenty of alternatives out there to learning SQL injection. This would include docker containers like damn vulnerable web application or even webgoat. What I like about this lab that I am creating is that we can see it every step of the way. This will allow us to have a deeper understanding of how it works behind the scenes. Especially considering I plan to cover methods used to harden, preventing or limiting SQL injection. That is it for today.
As always, it is important to Never Stop Learning!
References
mysqli_num_rows